I promise I'm not a Pokémon enthusiast, but the PokeAPI is just a good playground for testing and learning about APIs, with that let me try to show how to test the PokeAPI using Postman. In this blog post, I'll take you through the process of creating a simple test suite for the PokeAPI using Postman. Which should give you a nice foundation to go off and test many other APIs. We'll cover some basic API requests and tests to ensure the API is functioning as expected.
What is the PokeAPI?
I'm going to assume you've never read any of my other posts and have just arrived at Earth; The PokeAPI is a RESTful API that provides data about Pokémon, including information about their species, abilities, moves, and more. You can access the API documentation at https://pokeapi.co/docs.
Setting up Postman
Before diving into the tests, make sure you have Postman installed on your computer. You can download it from https://www.postman.com/downloads/. Once installed, open Postman and create a new collection to store your PokeAPI requests.
Test 1
To retrieve information about a specific Pokémon by ID or name, we'll use the following endpoint: https://pokeapi.co/api/v2/pokemon/{pokemon}
. Replace {pokemon}
with the ID or name of the Pokémon you're interested in.
For example: HTTP Request: GET URL: https://pokeapi.co/api/v2/pokemon/pikachu
To validate the response, we'll add the following tests:
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Response contains expected Pokémon data", function () {
const jsonData = pm.response.json();
pm.expect(jsonData.id).to.be.a('number');
pm.expect(jsonData.name).to.be.a('string');
pm.expect(jsonData.species).to.be.an('object');
pm.expect(jsonData.species.name).to.be.a('string');
});
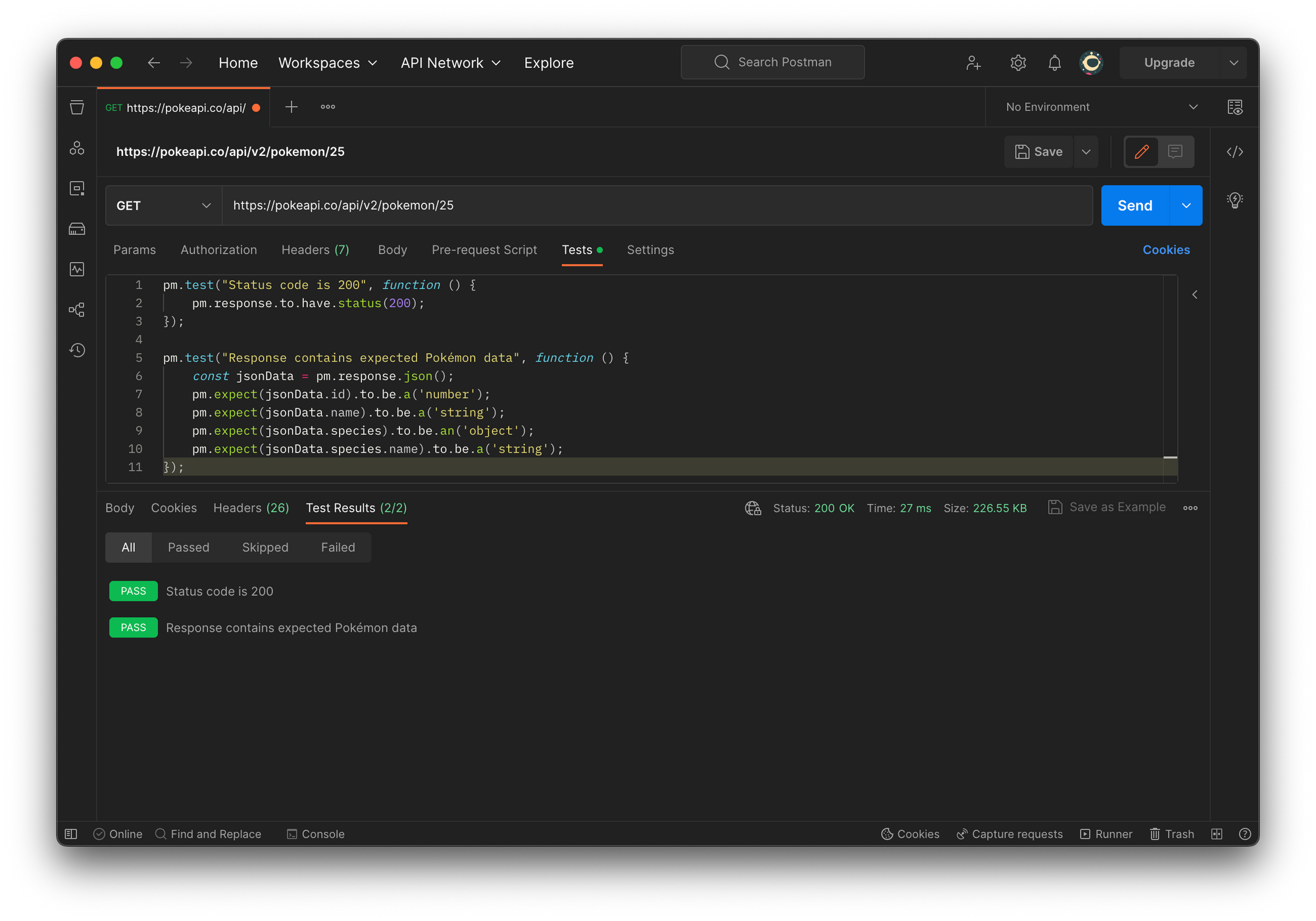
Test 2
To retrieve a list of Pokémon, you'll use the following endpoint: https://pokeapi.co/api/v2/pokemon
.
For example: HTTP Request: GET URL: https://pokeapi.co/api/v2/pokemon
Add the following tests to validate the response:
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Response contains a list of Pokémon", function () {
const jsonData = pm.response.json();
pm.expect(jsonData.results).to.be.an('array').that.is.not.empty;
});
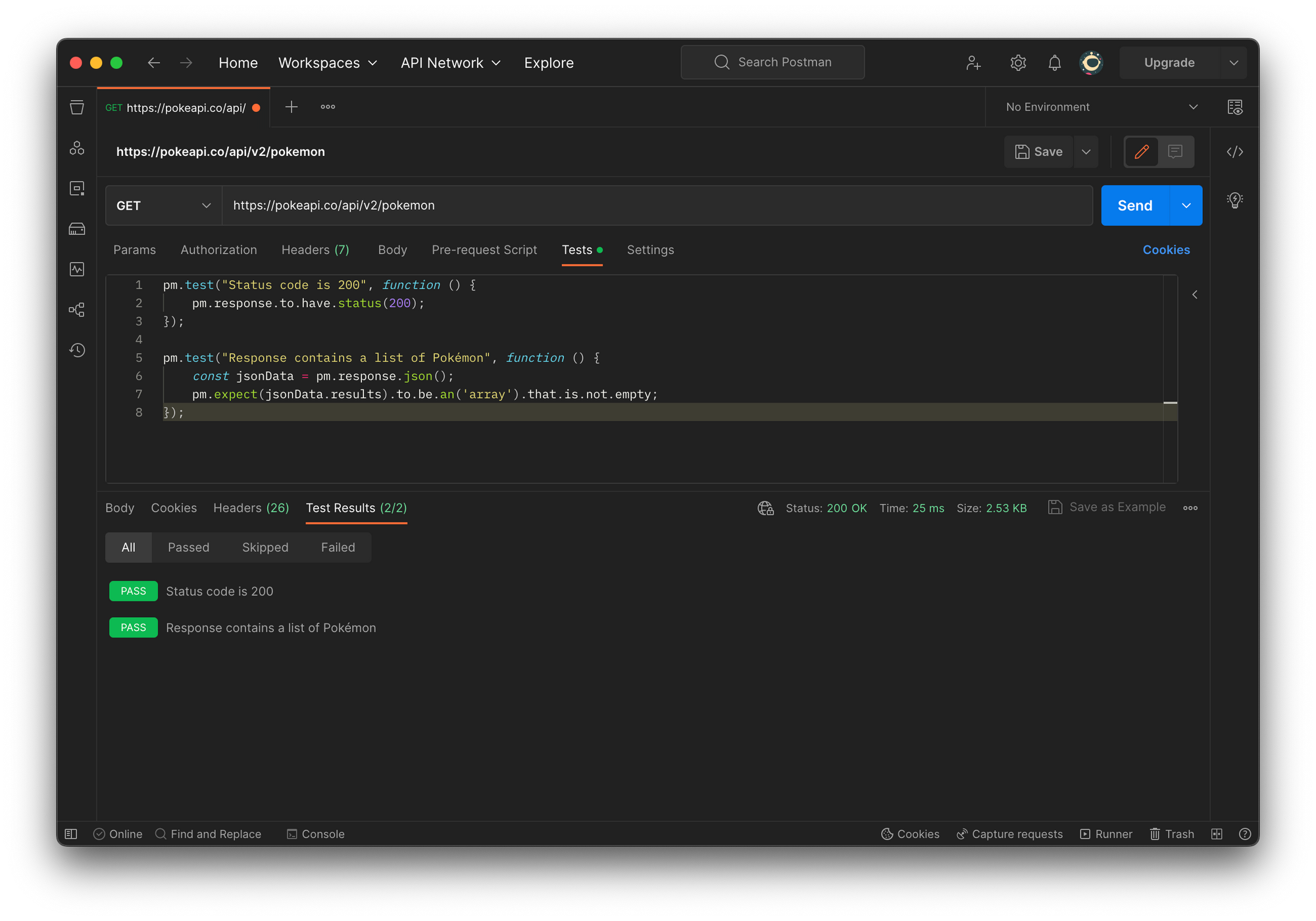
Test 3
To retrieve information about a specific ability by ID or name, use the following endpoint: https://pokeapi.co/api/v2/ability/{ability}
. Replace {ability}
with the ID or name of the ability you're interested in.
For example: HTTP Request: GET URL: https://pokeapi.co/api/v2/ability/stench
Add the following tests to validate the response:
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Response contains expected ability data", function () {
const jsonData = pm.response.json();
pm.expect(jsonData.id).to.be.a('number');
pm.expect(jsonData.name).to.be.a('string');
pm.expect(jsonData.effect_entries).to.be.an('array').that.is.not.empty;
});
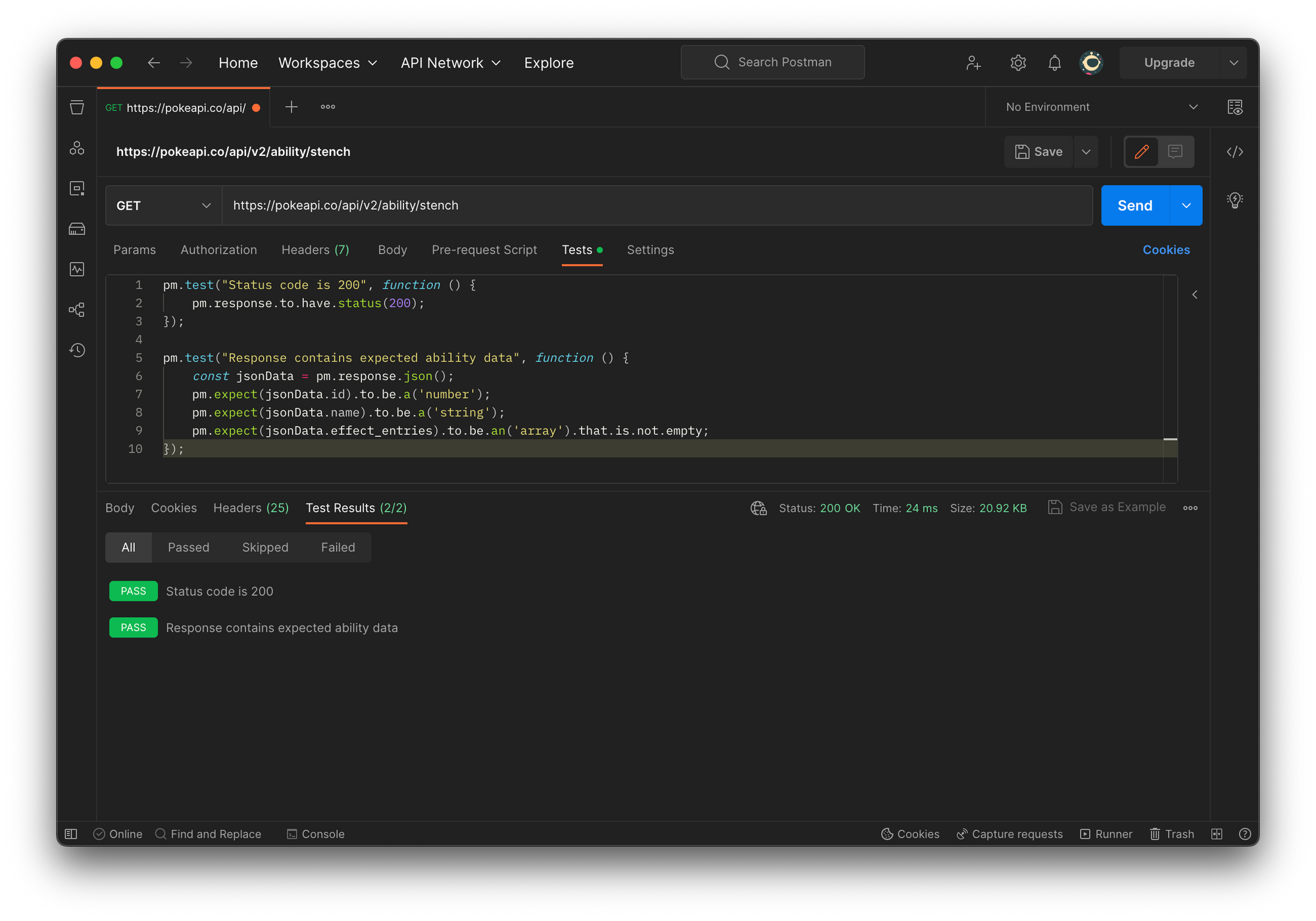
Test 4
To retrieve a list of abilities, use the following endpoint: https://pokeapi.co/api/v2/ability
.
For example: HTTP Request: GET URL: https://pokeapi.co/api/v2/ability
Add the following tests to validate the response:
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
pm.test("Response contains a list of abilities", function () {
const jsonData = pm.response.json();
pm.expect(jsonData.results).to.be.an('array').that.is.not.empty;
});
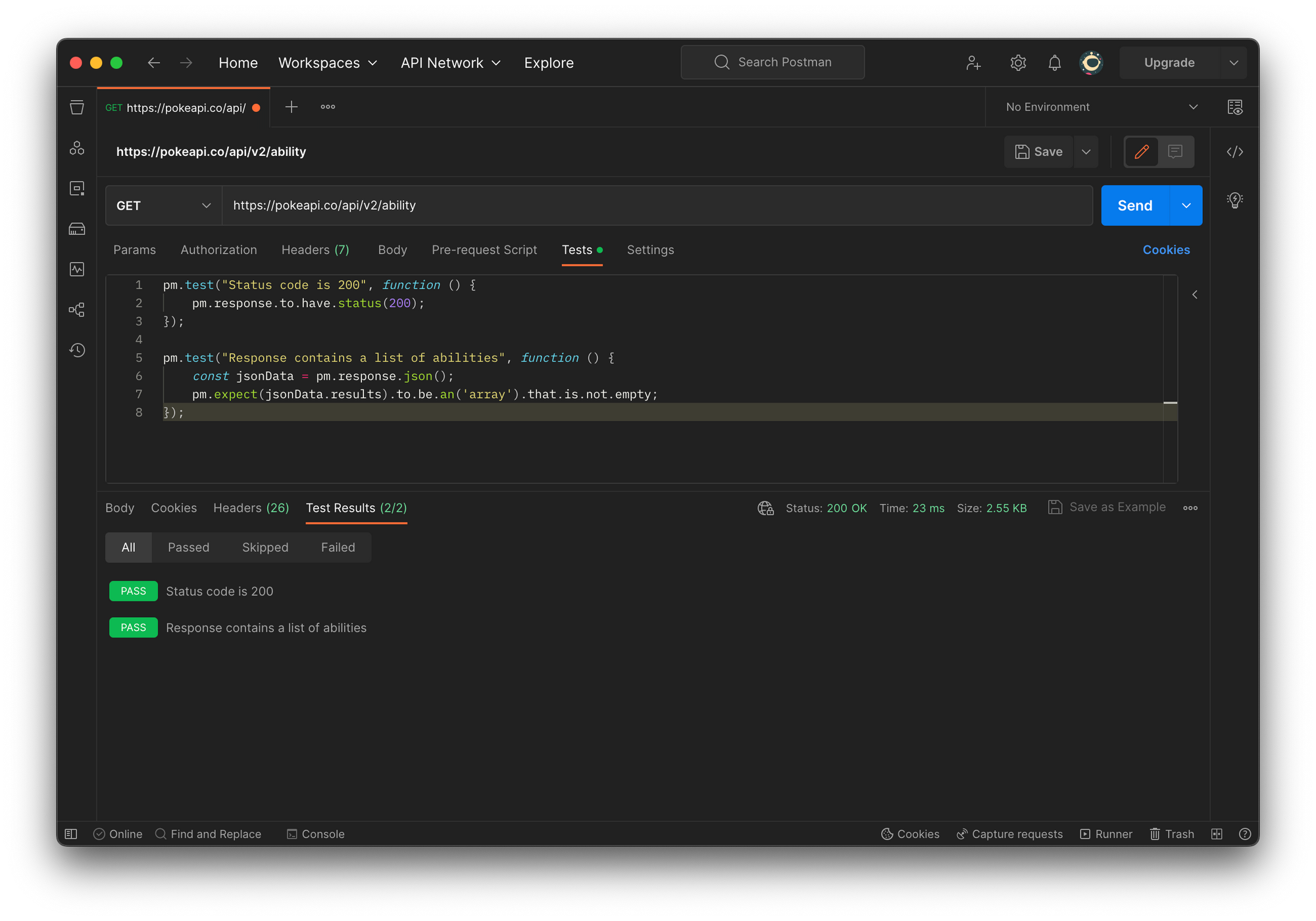
Hopefully, you can now create a simple test suite for the PokeAPI using Postman. The tests cover basic API requests and ensure that the API is functioning correctly. You can extend this test suite by adding more tests for different endpoints, checking edge cases, and even incorporating performance testing for more comprehensive analysis. I'll potentially cover that in a future post!